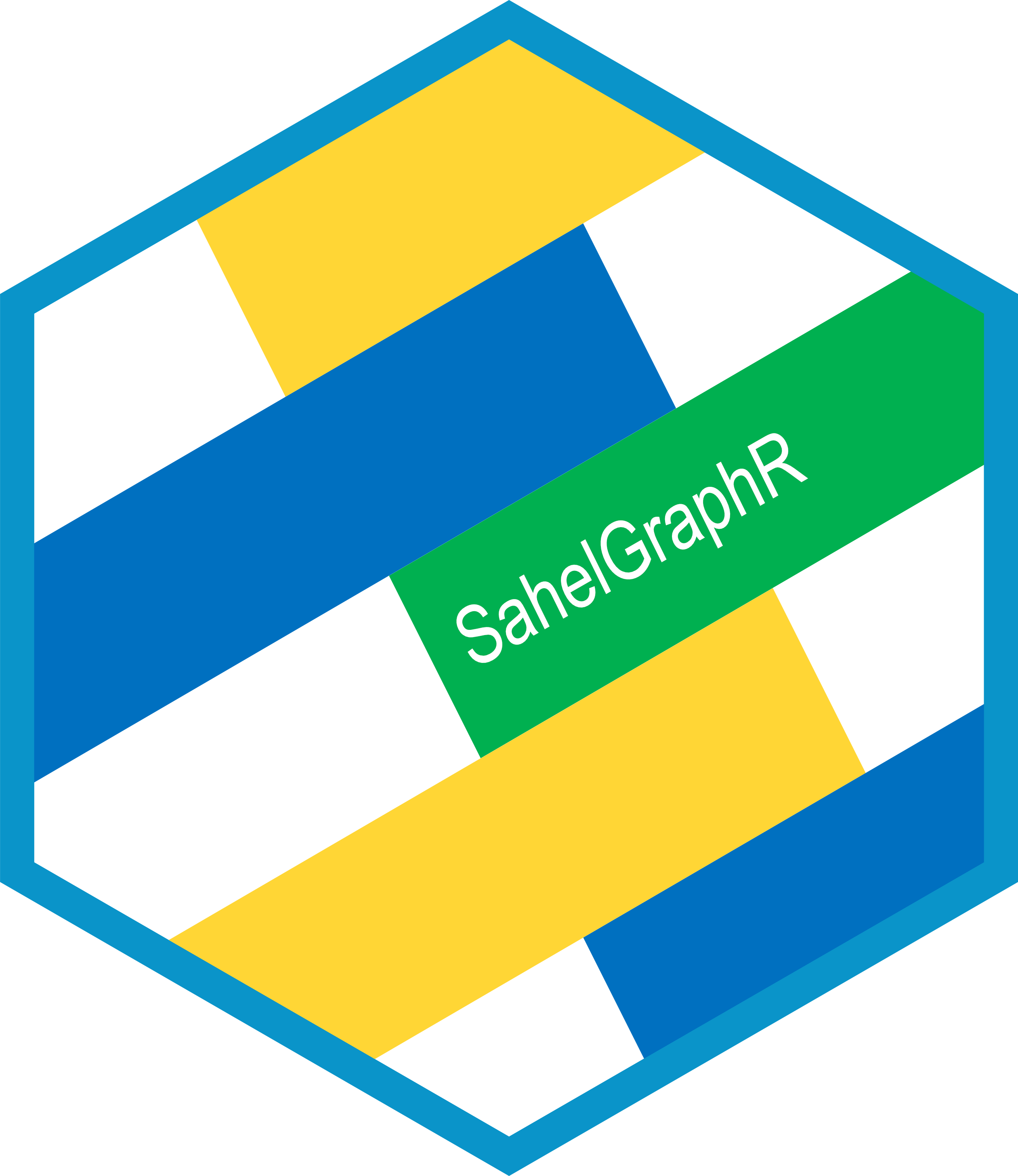
Collate predicted values of a list of models into a large data frame.
collate_predictions.Rd
Collate predicted values of a list of models into a large data frame.
Arguments
- models
List of regression models.
- by
String of the variable name to take the mean prediction by.
NULL
by default.
Examples
# Generate an example dataset
swiss2 <- dplyr::bind_cols(swiss,
treatment = rbinom(seq(nrow(swiss)),1, 0.5)) |>
dplyr::mutate(Education = Education + 2.5 * treatment)
reg1 <- lm(formula = Education ~ treatment, swiss2)
reg2 <- lm(formula = Education ~ treatment + Catholic, swiss2)
reg3 <- lm(formula = Education ~ treatment * Catholic, swiss2)
models <- list(reg1,
reg2,
reg3)
# Full predictions:
collate_predictions(models = models)
#> # A tibble: 141 × 11
#> modelID .rownames Education treatment .fitted .resid .hat .sigma .cooksd
#> <int> <chr> <dbl> <int> <dbl> <dbl> <dbl> <dbl> <dbl>
#> 1 1 Courtelary 12 0 11.3 0.679 0.0357 9.82 9.37e-5
#> 2 1 Delemont 9 0 11.3 -2.32 0.0357 9.82 1.10e-3
#> 3 1 Franches-Mnt 7.5 1 13.0 -5.47 0.0526 9.79 9.31e-3
#> 4 1 Moutier 7 0 11.3 -4.32 0.0357 9.80 3.80e-3
#> 5 1 Neuveville 15 0 11.3 3.68 0.0357 9.81 2.75e-3
#> 6 1 Porrentruy 9.5 1 13.0 -3.47 0.0526 9.81 3.75e-3
#> 7 1 Broye 9.5 1 13.0 -3.47 0.0526 9.81 3.75e-3
#> 8 1 Glane 8 0 11.3 -3.32 0.0357 9.81 2.25e-3
#> 9 1 Gruyere 9.5 1 13.0 -3.47 0.0526 9.81 3.75e-3
#> 10 1 Sarine 13 0 11.3 1.68 0.0357 9.82 5.74e-4
#> # ℹ 131 more rows
#> # ℹ 2 more variables: .std.resid <dbl>, Catholic <dbl>
# Grouped by model and treatment status:
collate_predictions(models = models, by = "treatment")
#> # A tibble: 6 × 3
#> modelID treatment mean_fitted_value
#> <int> <int> <dbl>
#> 1 1 0 11.3
#> 2 1 1 13.0
#> 3 2 0 11.3
#> 4 2 1 13.0
#> 5 3 0 11.3
#> 6 3 1 13.0